With the next release of Stellar Crossroads, I am planning on including a space combat simulation into the game. I know that I will be able implement something, however with some guidance, what I am able to achieve will be increased. I have several months to work out a solution, however raising now such that I don't get off on the wrong foot.
Below is the project folder available on Mega for the mini game in development. It's fully automated to update whenever I update the mini game code.
Ren'Py Project Folder -
I realise the limitations of Ren'Py, hence plan is for a 2D top down simulation with about a dozen ships on each side. Player would just assign fairly simply overall tactics to different wings of their force. The combat would then run through a turn using initiative sequence. Each ship would then target enemy based upon tactics and chances to hit and damage would be applied should the shot hit.
The first initial challenge is to determine how the ship information will be stored. Thus I require a data set for the fleet that will achieve the following:
- Be able to add and subtract ships with their data from the fleet.
- Able to count the number of ships in that fleet.
- Have a series of variables for a ship containing such information as weapons type, armour type, evasion, hit points etc.
- Individual items of data within the set must be accessible and also easily changed.
I have used data arrays in a format such as follows, however is this the best option?
At this stage thinking that a fleet will contain up to 16 ships. There will be multiple fleets and also a hangar to store more ships. It will need to be possible to transfer a ship from one location to another.
Just the data question initially, however more will follow as I develop the system.
PS: To assure you that assistance won't be in vain, a little screen below of the refining system I put into the game about a year ago. There is also mining and manufacturing in the game already. I'll get something implemented.
Below is the project folder available on Mega for the mini game in development. It's fully automated to update whenever I update the mini game code.
Ren'Py Project Folder -
You must be registered to see the links
I realise the limitations of Ren'Py, hence plan is for a 2D top down simulation with about a dozen ships on each side. Player would just assign fairly simply overall tactics to different wings of their force. The combat would then run through a turn using initiative sequence. Each ship would then target enemy based upon tactics and chances to hit and damage would be applied should the shot hit.
The first initial challenge is to determine how the ship information will be stored. Thus I require a data set for the fleet that will achieve the following:
- Be able to add and subtract ships with their data from the fleet.
- Able to count the number of ships in that fleet.
- Have a series of variables for a ship containing such information as weapons type, armour type, evasion, hit points etc.
- Individual items of data within the set must be accessible and also easily changed.
I have used data arrays in a format such as follows, however is this the best option?
Python:
l_fleet = {
1:
{ 1: "Bomber", 2: "Missile", 3: 5, 4: 50, 5: 1000},
2:
{ 1: "Fighter", 2: "Laser", 3: 10, 4: 20, 5: 800},
}
Just the data question initially, however more will follow as I develop the system.
PS: To assure you that assistance won't be in vain, a little screen below of the refining system I put into the game about a year ago. There is also mining and manufacturing in the game already. I'll get something implemented.
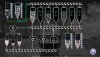
Last edited: